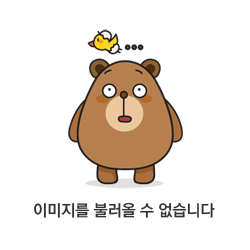
1. Create New Android Project
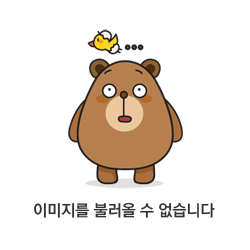
kotlin으로 진행하는 줄 알았는데 java로 만드는 안드로이드 ...!
그래도 안드로이드 앱 개발할때 kotlin이랑 java 파일 섞어도 된다고 한다.
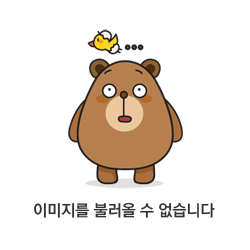
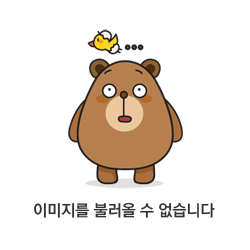
이거 두개 복사해서 build.gradle에 추가
2. Configure Retrofit for REST API Calls
안드로이드 스튜디오에 Employee 갖다붙이기
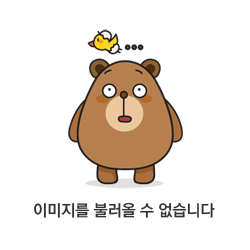
Employee.java
package com.example.springclient.model;
public class Employee {
private int id;
private String name;
private String location;
private String branch;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getLocation() {
return location;
}
public void setLocation(String location) {
this.location = location;
}
public String getBranch() {
return branch;
}
public void setBranch(String branch) {
this.branch = branch;
}
@Override
public String toString() {
return "Employee{" +
"id=" + id +
", name='" + name + '\'' +
", location='" + location + '\'' +
", branch='" + branch + '\'' +
'}';
}
}
RetrofitService.java
package com.example.springclient.retrofit;
import com.google.gson.Gson;
import retrofit2.Retrofit;
import retrofit2.converter.gson.GsonConverterFactory;
public class RetrofitService {
private Retrofit retrofit;
public RetrofitService() {
initializeRetrofit();
}
private void initializeRetrofit() {
//give the address of the server where springboot is running
retrofit = new Retrofit.Builder()
.baseUrl("http://your-ip-address:9000")
.addConverterFactory(GsonConverterFactory.create(new Gson()))
.build();
}
public Retrofit getRetrofit() {
return retrofit;
}
}
convertfactory : 우리가 json schema를 사용하므로 convert가 필요해서
EmployeeApi.java
package com.example.springclient.retrofit;
import com.example.springclient.model.Employee;
import java.util.List;
import retrofit2.Call;
import retrofit2.http.Body;
import retrofit2.http.GET;
import retrofit2.http.POST;
public interface EmployeeApi {
@GET("/employee/get-all")
Call<List<Employee>> getAllEmployees();
@POST("/employee/save")
Call<Employee> save(@Body Employee employee);
}
3. Design&Code the Employee creation form
activity_main.xml 코드 생략
5. Making POST request via Retrofit
MainActivity.java
package com.example.springclient;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.Toast;
import com.example.springclient.model.Employee;
import com.example.springclient.retrofit.EmployeeApi;
import com.example.springclient.retrofit.RetrofitService;
import com.google.android.material.button.MaterialButton;
import com.google.android.material.textfield.TextInputEditText;
import java.util.logging.Level;
import java.util.logging.Logger;
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initializeComponents();
}
private void initializeComponents() {
TextInputEditText inputEditTextName = findViewById(R.id.form_textFieldName);
TextInputEditText inputEditBranch = findViewById(R.id.form_textFieldBranch);
TextInputEditText inputEditLocation = findViewById(R.id.form_textFieldLocation);
MaterialButton buttonSave = findViewById(R.id.form_buttonSave);
RetrofitService retrofitService = new RetrofitService();
EmployeeApi employeeApi = retrofitService.getRetrofit().create(EmployeeApi.class);
buttonSave.setOnClickListener(view -> {
String name = String.valueOf(inputEditTextName.getText());
String branch = String.valueOf(inputEditBranch.getText());
String location = String.valueOf(inputEditLocation.getText());
Employee employee = new Employee();
employee.setName(name);
employee.setBranch(branch);
employee.setLocation(location);
employeeApi.save(employee)
.enqueue(new Callback<Employee>() {
@Override
public void onResponse(Call<Employee> call, Response<Employee> response) {
Toast.makeText(MainActivity.this, "Save successful!", Toast.LENGTH_SHORT).show();
}
@Override
public void onFailure(Call<Employee> call, Throwable t) {
Toast.makeText(MainActivity.this, "Save failed!!!", Toast.LENGTH_SHORT).show();
Logger.getLogger(MainActivity.class.getName()).log(Level.SEVERE, "Error occurred", t);
}
});
});
}
}
enqueue : sending data throught the network can take some time so the application should not like be unresponsive or anything when that happens
-> 메인 스레드에서 동작하지 않을 수 있으니 enqueue function을 이용해서 시간 단축? 하튼 돌아가게 한다는 뜻
AndroidManifest.xml
두개 퍼미션 추가
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
다 하고 실행해봤더니
An issue was found when checking AAR metadata:
1. Dependency 'androidx.activity:activity:1.8.0' requires libraries and applications that
depend on it to compile against version 34 or later of the
Android APIs.
:app is currently compiled against android-33.
Recommended action: Update this project to use a newer compileSdk
of at least 34, for example 34.
Note that updating a library or application's compileSdk (which
allows newer APIs to be used) can be done separately from updating
targetSdk (which opts the app in to new runtime behavior) and
minSdk (which determines which devices the app can be installed
on).
뭐 이런 오류 떠서 찾아봤더니 레벨을 올려야된다고 한다.
지피티가 알려준대로 ..
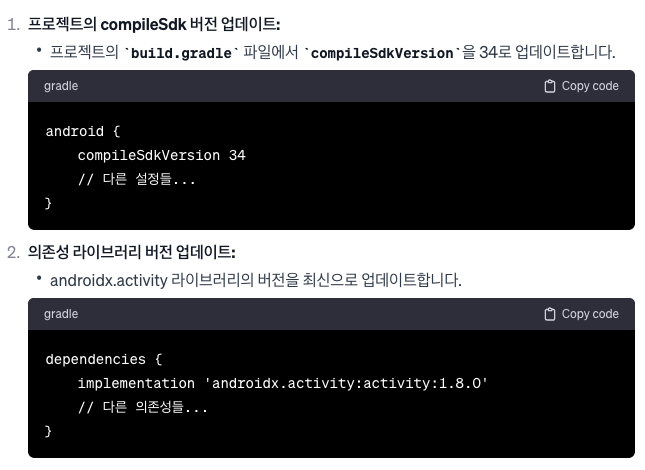
요로코롬 바꿨더니 잘된다!
매니페스트 파일에 하나 더 추가한 것
android:usesCleartextTraffic="true"
우리가 샘플 프로젝트로 하고있기때문에 https에 ip주소를 넣고 ? 보안성문제로 원래 안된다고 한다
그걸 무시하고 일단 무시하고 진행하겠다는 뜻인것같다. 나중에 배포할때는 다르게 하는 방법 공부해야할듯
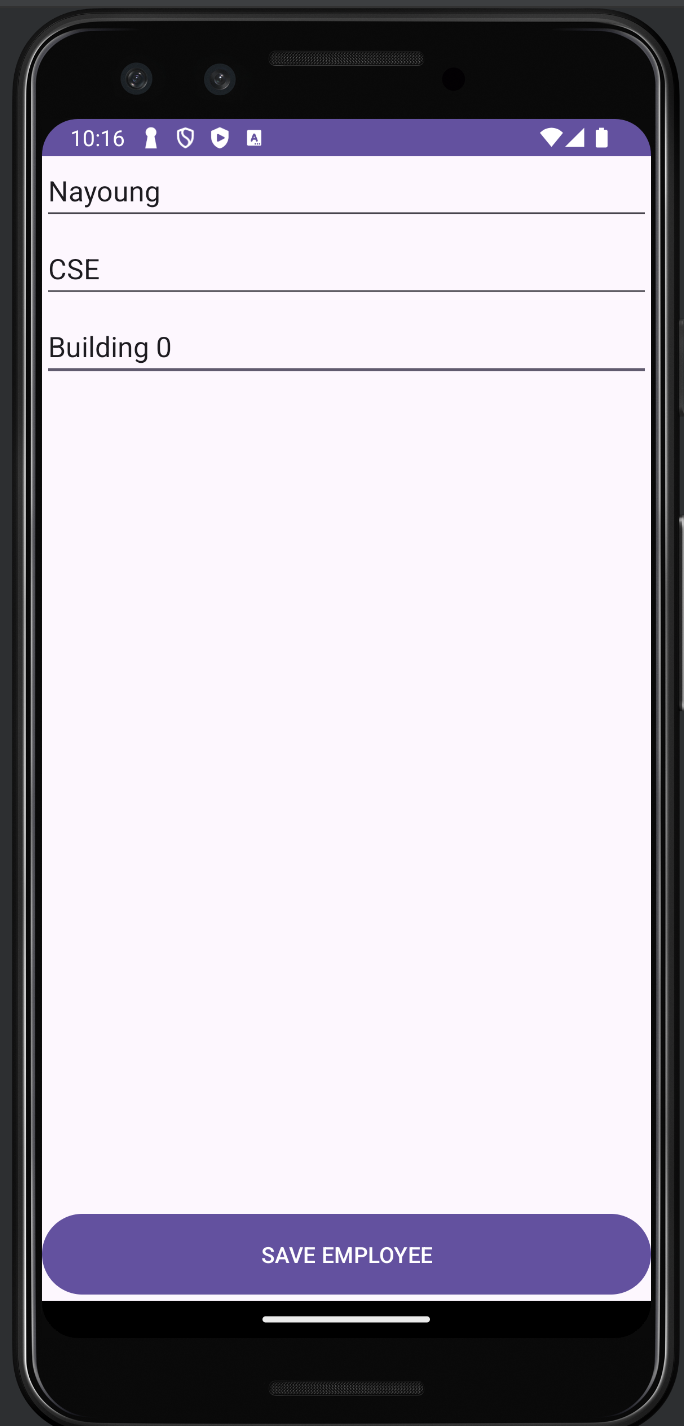
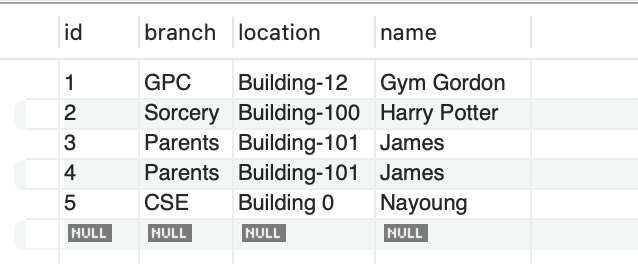
mysql에서 저장된 것 확인완료~!!!!! 뿌듯하다
하나 또 빼먹었던 건 SpringBoot 프로젝트에서 BootRun 실행한 상태로 돌려야된다!
'BackEnd : Spring > SpringBoot' 카테고리의 다른 글
Android + Spring Boot + Firebase Google Login - FE (1) | 2024.01.25 |
---|---|
Spring Boot Server & Android Client App - Chapter #5, #6 (0) | 2024.01.14 |
Spring Boot Server & Android Client App - Chapter #3 (0) | 2024.01.14 |
Spring Boot Server & Android Client App - Chapter #2 (0) | 2024.01.13 |
Spring Boot Server & Android Client App - Chapter #1 (0) | 2024.01.12 |